I’m trying to load an image from an URL and then apply it to a GTKImage, however, I’m running under some issues.
let url = element.attributes.get("src").unwrap().clone().unwrap();
let handle = thread::spawn(move || {
let result = reqwest::blocking::get(url).unwrap().bytes().unwrap();
result
});
let img_data = handle.join().unwrap();
let img_stream = gio::MemoryInputStream::from_bytes(&Bytes::from(&img_data));
let stream =
gdk_pixbuf::Pixbuf::from_stream(&img_stream, Some(&gio::Cancellable::new()))
.unwrap();
let image = gtk::Image::builder()
.css_name("img")
.css_classes(element.classes.clone())
.pixel_size(stream.height())
.halign(gtk::Align::Start)
.build();
image.set_from_pixbuf(Some(&stream));
html_view.append(&image);
My problem is that the normal width & height of the image (112x140 in this case) don’t correctly apply (because the image has “GTK_ICON_SIZE_INHERIT”, and icon_size(...)
only accepts an enum
with 3 values: Inherit
, Small
, Large
). I resulted into setting the image semi-properly using pixel_size()
of width()
, but that means it won’t properly check for the height.
Images below for reference
Testing https://i.pinimg.com/originals/25/aa/1a/25aa1a16b78d63f639b04b8427d4f2d6.jpg
:
Testing
https://i.pinimg.com/originals/25/aa/1a/25aa1a16b78d63f639b04b8427d4f2d6.jpg
, but without modifying pixel_size
(the default behavior, and where the problem is):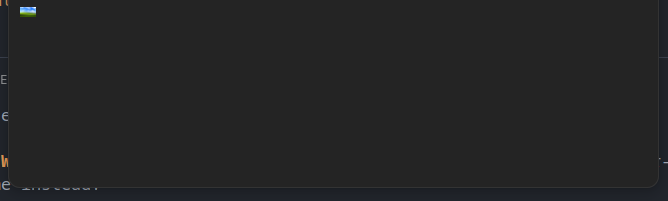
And the confusing part is that the width()
and height()
on the PixBuf is correct, it’s the way GTKImage “renders it” that probably makes it small.
I’m confused - what’s the proper way of doing this?